Why do variables not change their values after being called by a function in C++? - Quora
Why do variables not change their values after being called by a function in C++?
Answer (1 of 4): I think I know what you are asking. Variables are not called by a function, they are passed to a function. There are three basic ways to do that: int F( int x) - when you call this function a copy of your variable is placed on the stack (o
www.quora.com
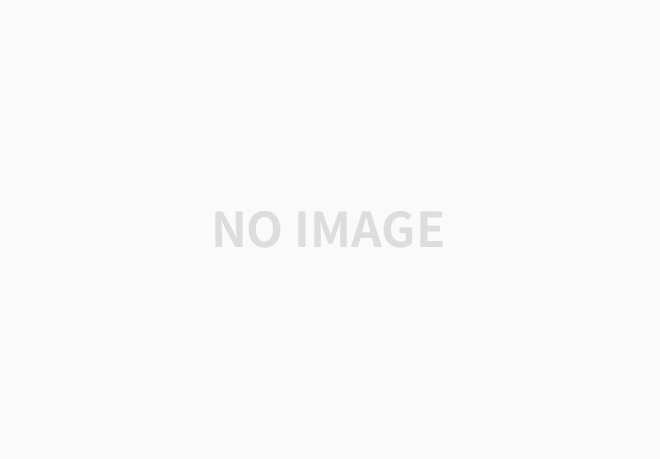
Why do variables not change their values after being called by a function in C++?
C++에서 함수 호출 후에도 변수의 값이 변하지 않는 이유는 무엇인가요?
Because their values weren’t changed.
No code was provided, so I am guessing you are talking about some case where a local variable with the same name is changed. Such as:
<c++ />void f(int a) { a=0; // sets the local variable a (at line 1) to 0. } … int a = 1; cout << a << endl; f(a); // prints the value of a in scope which is set to 1 at line 5. cout << a << endl;
Output:
<c++ />1 1
Now if you want the value to change you should have passed by reference.
<c++ />void f(int& a) { // a is a reference a=0; // sets the referenced value to 0. } … int a = 1; cout << a << endl; f(a); // prints the value of a in scope. // The value has been modified by reference at line 2. cout << a << endl;
Output:
<c++ />1 0
Variables in C++ are either passed by value or passed by reference (&)
If you pass by value, you are saying make a separate copy of the variable and that will be sent to the functions. Any changes you make there are only in the copy and not reflected in the calling function.
If you pass by reference, you are passing the address of the variable to function, so any changes made in the function are reflected back in the calling function.
int F( int x) - when you call this function a copy of your variable is placed on the stack (or, likely in a register). The function messes with that copy. Perhaps it returns the modified value. Did you store it? If not, your variable is not changed. A copy of it was passed and modified.
The above is pass by value. The other two ways are pass by reference. In each of them, the address of your variable is passed to the function and the function manipulates your variable. This is useful when the variable is a large array or structure.
Void f( int *x) passes a pointer to your variable. The function must dereference the pointer to access your variable. The function can see and even modify the address held in the pointer.
Void f(int &x) passes a pointer to your variable, but this time it's called a “reference”. The called function treats it as a variable, C++ handles the pointer dereference for you. The function can't modify or even see the address held in the reference.
Both these methods will change your variable.
'컴공' 카테고리의 다른 글
[C++] 오버로드와 템플릿 (Overloads and templates) (0) | 2022.03.12 |
---|---|
OpenGL 비주얼 스튜디오 .dll 링킹 관련 오류 (glew와 freeglut 라이브러리 링킹 오류) (0) | 2021.12.01 |
[C++] stringstream (0) | 2021.08.22 |
[C++] 프로그래밍 할 때 클래스를 사용하는 이유 (0) | 2021.08.20 |
Lecture 5 : Scheduling and Binary Search Trees (0) | 2021.07.23 |